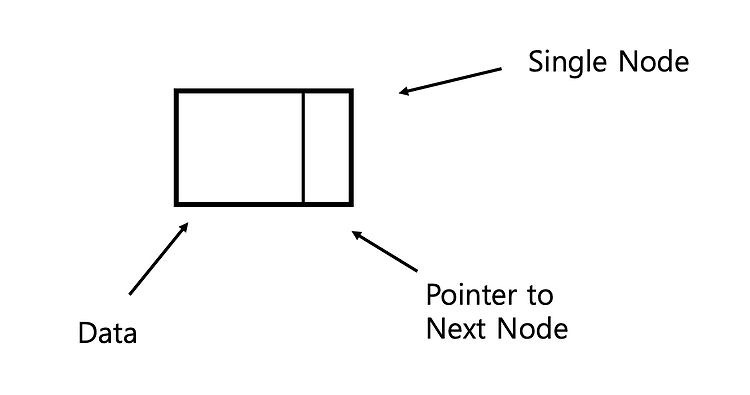
Linked List
·
CS/Data Structure
Definition of Linked ListConsists of NodesNode: unit of storage (item)Nodes are linked with pointers(link) - 포인터가 옆에 붙어있다 (what does that mean?)메모리 아무 곳에나 흩어져있음논리적으로는 1차원pointer to next node : 단순히 주소가 저장되어 있는 것Q. 화살표는 코드에서 무슨 뜻일까? A. 다음 노드의 주소가 값으로 적혀있다화살표를 따라가면 다음 노드가 어디에 있는지 알 수 있다 프로그램의 head는 변수로 가지고 있어야한다 - head는 노드가 아니다Code of nodeclass Node { int a; Node *n;};Q. node 옆에 *이 없다면 어떻게 될까A. 노드가..