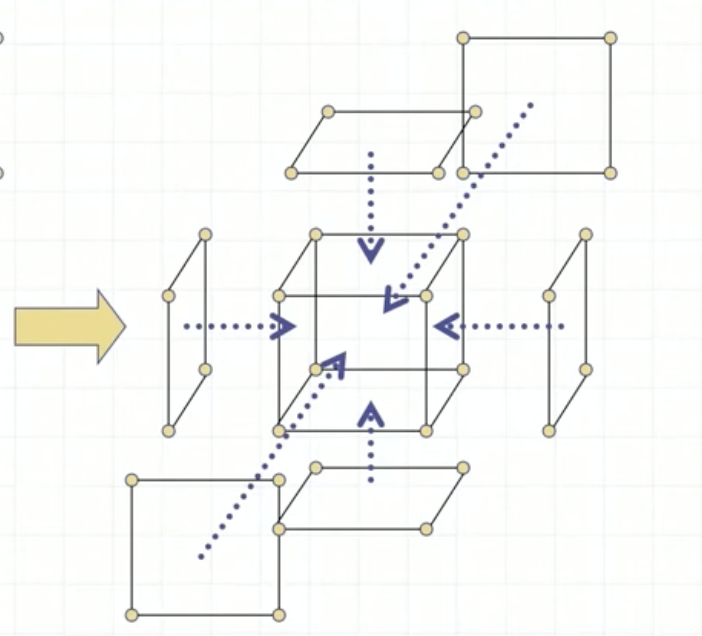
Graph (Subgraphs, Unions, Isomorphism, Path)
·
CS/Data Structure
Subgraphs그래프의 일부 Defintion Let G = (V,E) and H = (W,F) be graphs. H is said to be a subgraph of G, if \(W\subseteq V\) and \(F\subseteq E\)G와 H가 모두 그래프이고, W가 V의 부분집합, F가 E의 부분 집합일 때 subgraph라고 한다 Q.How many \(Q_2\) subgraphs does \(Q_3\) have?A. 같다의 약한 개념에서는 6, 강한 개념에서는 1개이다노드에 번호를 부여하였을 때, 노드의 번호가 다르다면, 다른 그래프이기 때문에 \(Q_2\)에 1,2,3,4라는 노드 번호와 일치해야한다. Unions강하게 같다 라는 개념을 사용하여 Q.How many \(Q_2\) s..