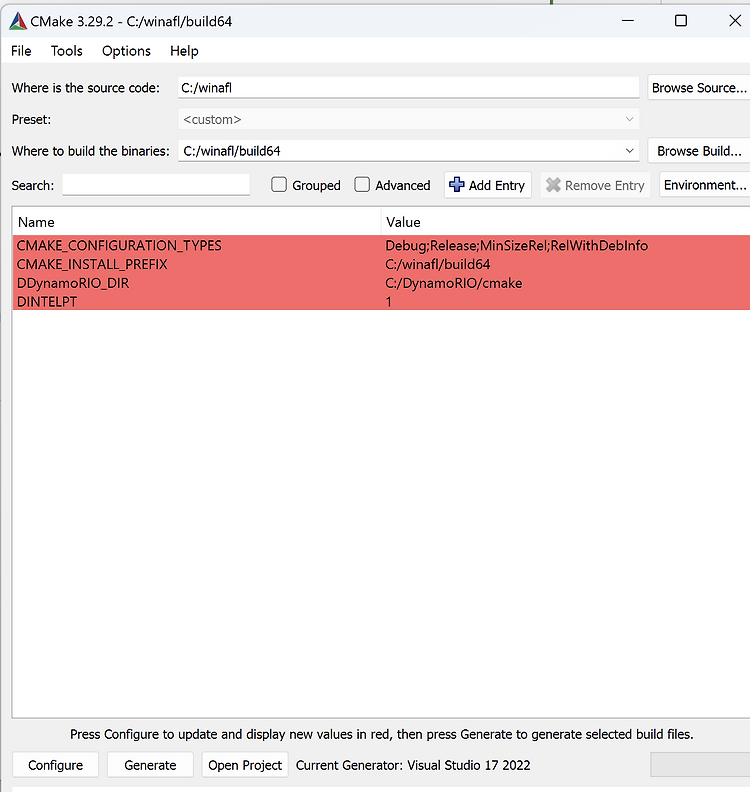
[Fuzzing] WinAFL을 이용한 개념정리
·
Hacking/Window
Definition | 정의Fuzz testing or fuzzing is an automated software testing method that injects invalid, malformed, or unexpected inputs into a system to reveal software defects and vulnerabilities. A fuzzing tool injects these inputs into the system and then monitors for exceptions such as crashes or information leakage. Put more simply, fuzzing introduces unexpected inputs into a system and watche..